利用双指针思想解决有序数组的平方排序问题
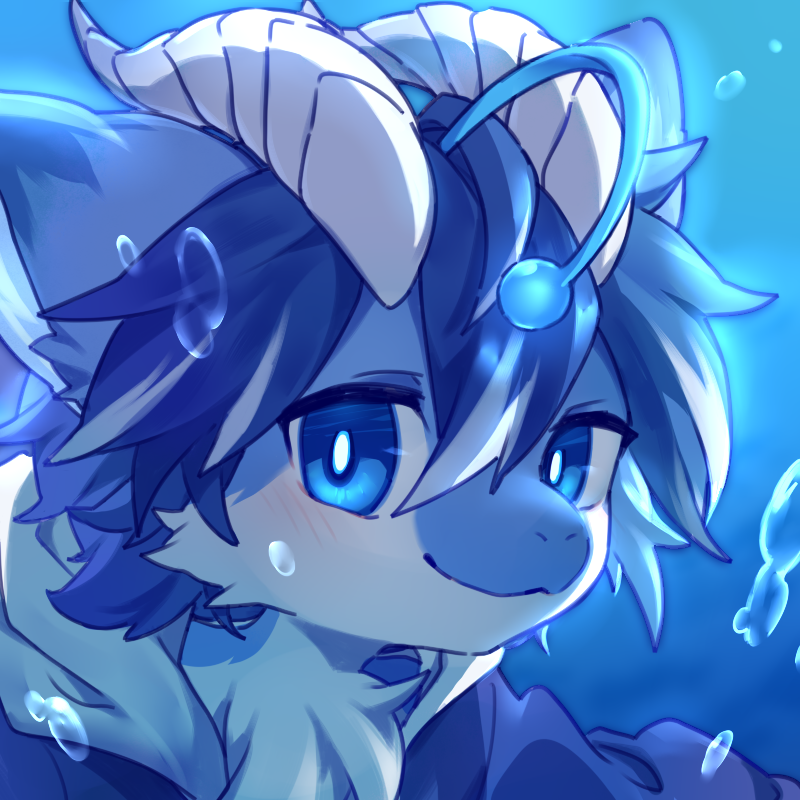
Question
当前存在一个以非递减顺序排序的整数数组nums
,编写算法返回每个数字的平方所组成的新数组,且同样按照非递减顺序排序。
本题最简单的思路即对每个数组元素均平方后的数组进行再排序,其时间复杂度为
以数列[-7, -3, 2, 3, 11]
为例,首先定义两个指针,其中负数指针low
指向nums
数组起始位置(即最小值位置),正数指针high
指向nums
数组末尾位置(即最大值位置)。再定义一个辅助新数组result
生成的索引指针index
,并指向result
数组的末尾位置。
1 | int* result = malloc(sizeof(int) * numsSize); |
当high
所指元素的平方结果大于low
所指元素的平方结果时,将high
所指元素的平方结果导入result
中index
所指位置。同时,high
左移至nums
的下一位并准备与当前low
所指元素进行比较,index
左移至result
的下一位。
1 | if(nums[low] * nums[low] < nums[high] * nums[high]){ |
当low
所指元素的平方结果大于high
所指元素的平方结果时,将low
所指元素的平方结果导入result
中index
所指位置。同时,low
右移至nums
的下一位并准备与当前high
所指元素进行比较,index
左移至result
的下一位。
1 | if(nums[low] * nums[low] > nums[high] * nums[high]){ |
故最终完整代码为
1 | int* sortedSquares(int* nums, int numsSize, int* returnSize){ |
Python下代码为
1 | class Solution(object): |
- Title: 利用双指针思想解决有序数组的平方排序问题
- Author: Zielorem
- Created at : 2023-01-08 11:42:16
- Updated at : 2023-07-14 01:05:16
- Link: https://zielorem.github.io/2023/01/08/square-sort/
- License: This work is licensed under CC BY-NC-SA 4.0.
Comments