数组的翻转与轮转
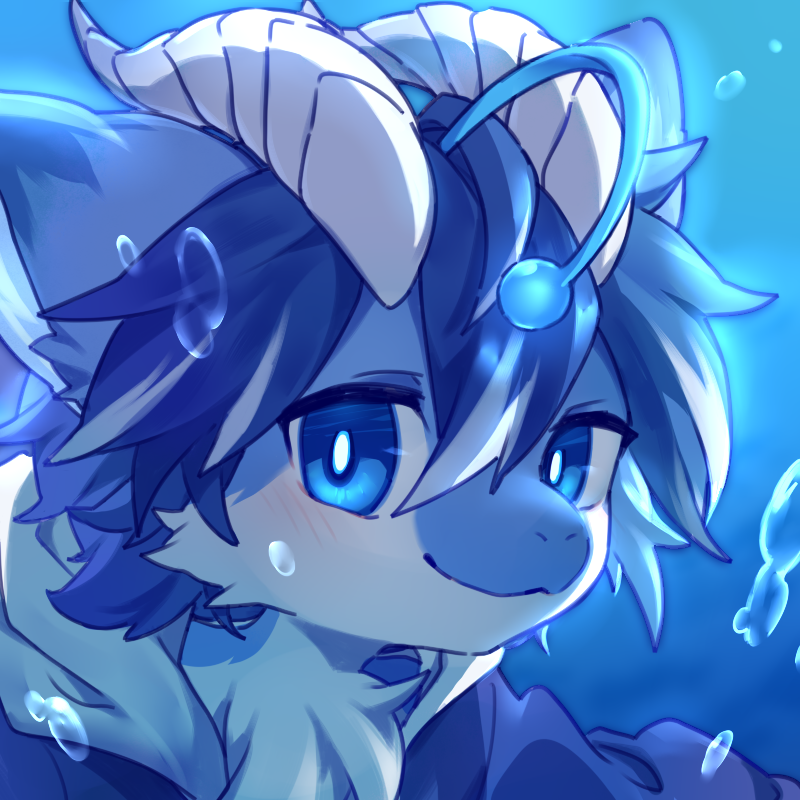
Question
当前存在一个数组nums
,编写算法将数组中的
为了避免直接轮转导致的数组元素覆盖,可以创建新的数组从而将中的元素存储至正确的位置,尔后再将其赋值至原数组中。在轮转过程中,当数组元素向右移动 [1, 2, 3, 4, 5, 6, 7]
为例,其轮转过程为
- 将所有数组元素翻转
- 翻转
区间内的元素
- 翻转
区间内的元素
翻转操作本质上为互换操作。因此,需要定义一个临时变量temp
用于替换操作。互换函数为
1 | void swap(int* a, int* b){ |
持有互换函数后,需在翻转函数中确定互换的元素对象。翻转函数为
1 | void reverse(int* nums, int low, int high){ |
因此,根据分析结果,轮转函数为
1 | void rotate(int* nums, int numsSize, int k){ |
完整代码为
1 | void swap(int* a, int* b){ |
Python下该算法代码为
1 | class Solution(object): |
更好的方法为通过slice
操作拼接数组
1 | class Solution(object): |
- Title: 数组的翻转与轮转
- Author: Zielorem
- Created at : 2023-01-08 14:47:42
- Updated at : 2025-05-30 12:39:26
- Link: https://zielorem.github.io/2023/01/08/rotate-array/
- License: This work is licensed under CC BY-NC-SA 4.0.
Comments