关于完美二叉树的指针填充策略
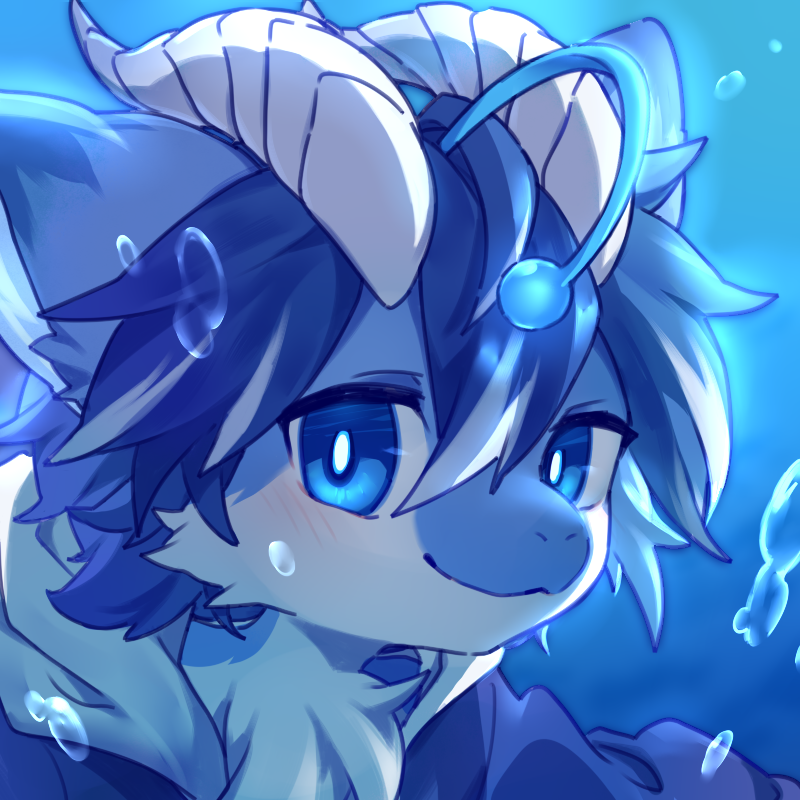
Question
给定一个完美二叉树,其所有叶子结点均在同一层,且每个父节点均有两个子节点,其定义为
1 | struct Node{ |
编写算法填充其每个next
指针,使该指针指向其下一个右侧节点。若不存在下一个右侧节点,则将next
指针设置为NULL
。缺省状态下,所有next
指针均被设置为NULL
。
通过分析完美二叉树的结构,可以发现位于该树每一层最右边的结点的next
指针均指向NULL
,且均为右节点或根结点。因此
- 若该层最左侧节点为空,则该层不存在
- 若某节点的父节点指向
NULL
,则该父节点的右节点一定指向NULL
因此,需要通过同时分析两层的状况以进行指针填充操作。即
- 父节点的左节点一定指向其右节点
- 当父节点的
next
指针不为空时,其右节点指向该父节点的next
指针所指向的节点的左节点 - 当父节点的
next
指针为空时,其右节点也指向NULL
首先,由于指针填充操作需要同时分析两层的状况,因此需要建立反映相邻两层状况的指针cur
与pre
1 | Node* cur = root->left->left; |
当根节点为空时,直接返回空指针,即
1 | if(root == nullptr){ |
当根节点不存在子结点时,直接返回根结点。由于该树为完美二叉树,因此若某节点的左节点或右节点不存在,则该节点的子结点均不存在
1 | if(root->left == nullptr){ |
对于某节点的左节点,其next
指针一定指向该结点的右节点,即
1 | if(root->left != nullptr){ |
对于某节点的右节点
- 若该右节点的父节点的
next
指针不为空,则该右节点指向其父节点的next
指针所指向的节点的左节点,即
1 | if(root->next != nullptr){ |
- 若该右节点的父节点的
next
指针为空,则该右节点的next
指针亦为空,即
1 | if(root->next == nullptr){ |
依据此逻辑,可以通过cur
指针与pre
指针对根结点的后续节点进行指针填充操作。由于父节点的左节点与右节点均存在,因此cur
指针需要沿着next
指针向右行进,并对该层其他的父节点执行操作。上文提出,若某层最左侧节点为空,则该层不存在。因此,操作的终止条件为cur
指针指向NULL
或pre
指针指向NULL
。
- 当
pre
指针指向NULL
时,所有cur
所在层的节点的父节点均遍历完毕。此时,需要将pre
指针与cur
指针同时推进至下一层,即
1 | pre = cur; |
- 当
cur
指针指向NULL
时,cur
指针所指节点的左节点不存在,即下一层所有节点均不存在。因此,当前cur
指针所在节点为叶子节点,cur
指针无法继续前行,整体遍历操作执行完毕
将上述代码段整合后,完整代码为
1 | class Solution{ |
Python下算法为
1 | class Solution: |
- Title: 关于完美二叉树的指针填充策略
- Author: Zielorem
- Created at : 2023-01-14 23:34:36
- Updated at : 2025-05-30 12:39:27
- Link: https://zielorem.github.io/2023/01/14/fill-pointer-of-trees/
- License: This work is licensed under CC BY-NC-SA 4.0.
Comments